为什么要线程池
之前实现的那个简陋的服务器只能接受一个连接,这显然是不行的,所以我们可以用多线程来解决这个问题
但是如果来一个连接就开一个线程那么连接很多的时候就会有开很多的线程,还是会影响性能,所以需要线程池,即固定最大可以开的线程,然后将线程管理起来,来一个连接就把它加入工作队列,然后呢让空闲的线程去竞争这个任务。
线程池实现
类定义
1 |
|
其中is_runing是原子变量,互斥锁和条件变量是来保证工作队列的访问的安全,同时该类是模板类,但是工作队列中的元素要保证是一个类,并且实现了一个run
函数
初始化
1 | template<class T> |
创建threadnum
个线程,线程的入口是本类的work函数
添加任务
1 | template<class T> |
队列不为空就唤醒一个线程
线程的入口函数
1 | template<class T> |
关闭线程池
1 | template<class T> |
测试
1 |
|
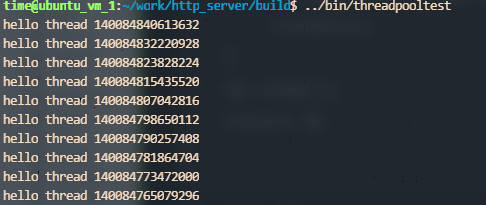